Accessing Argo data by float using Argopy#
Although the goal of the Argo Online school is to teach the basics of the Argo data, and therefore, we explain the data using the primary source, it is worth mentioning argopy:
is a python library dedicated to Argo data access, manipulation and visualisation for standard users as well as Argo experts. the Argo dataset is very complex: with thousands of different variables, tens of reference tables and a user manual more than 100 pages long: argopy aims to help you navigate this complex realm.
In this notebook we show a few examples, but we refer to the argopy Gallery for a more detailled explanation:
Fist, as usual, import the libraries:
import numpy as np
import matplotlib as mpl
from matplotlib import pyplot as plt
import xarray as xr
xr.set_options(display_expand_attrs = False)
<xarray.core.options.set_options at 0x10914fc50>
Import argopy and set-up a data fetcher:
import argopy
argopy.reset_options()
argopy.clear_cache()
from argopy import DataFetcher # This is the class to work with Argo data
from argopy.plot import scatter_map, scatter_plot # Functions to easily make maps and plots
You can load one profile from a float:
ArgoSet = DataFetcher().profile(6901254,1)
apProfile = ArgoSet.load().data
/Users/pvb/miniconda3/envs/AOS_clean/lib/python3.12/site-packages/argopy/xarray.py:70: FutureWarning: The return type of `Dataset.dims` will be changed to return a set of dimension names in future, in order to be more consistent with `DataArray.dims`. To access a mapping from dimension names to lengths, please use `Dataset.sizes`.
self._dims = list(xarray_obj.dims.keys())
/Users/pvb/miniconda3/envs/AOS_clean/lib/python3.12/site-packages/argopy/xarray.py:70: FutureWarning: The return type of `Dataset.dims` will be changed to return a set of dimension names in future, in order to be more consistent with `DataArray.dims`. To access a mapping from dimension names to lengths, please use `Dataset.sizes`.
self._dims = list(xarray_obj.dims.keys())
/Users/pvb/miniconda3/envs/AOS_clean/lib/python3.12/site-packages/argopy/xarray.py:70: FutureWarning: The return type of `Dataset.dims` will be changed to return a set of dimension names in future, in order to be more consistent with `DataArray.dims`. To access a mapping from dimension names to lengths, please use `Dataset.sizes`.
self._dims = list(xarray_obj.dims.keys())
/Users/pvb/miniconda3/envs/AOS_clean/lib/python3.12/site-packages/argopy/xarray.py:70: FutureWarning: The return type of `Dataset.dims` will be changed to return a set of dimension names in future, in order to be more consistent with `DataArray.dims`. To access a mapping from dimension names to lengths, please use `Dataset.sizes`.
self._dims = list(xarray_obj.dims.keys())
apProfile
<xarray.Dataset> Size: 18kB Dimensions: (N_POINTS: 148) Coordinates: * N_POINTS (N_POINTS) int64 1kB 0 1 2 3 4 5 ... 143 144 145 146 147 LATITUDE (N_POINTS) float64 1kB 29.16 29.16 29.16 ... 29.18 29.18 LONGITUDE (N_POINTS) float64 1kB -15.49 -15.49 ... -15.43 -15.43 TIME (N_POINTS) datetime64[ns] 1kB 2018-10-23T20:54:00 ... 20... Data variables: (12/15) CYCLE_NUMBER (N_POINTS) int64 1kB 1 1 1 1 1 1 1 1 1 ... 1 1 1 1 1 1 1 1 DATA_MODE (N_POINTS) <U1 592B 'R' 'R' 'R' 'R' 'R' ... 'R' 'R' 'R' 'R' DIRECTION (N_POINTS) <U1 592B 'D' 'D' 'D' 'D' 'D' ... 'A' 'A' 'A' 'A' PLATFORM_NUMBER (N_POINTS) int64 1kB 6901254 6901254 ... 6901254 6901254 POSITION_QC (N_POINTS) int64 1kB 1 1 1 1 1 1 1 1 1 ... 1 1 1 1 1 1 1 1 PRES (N_POINTS) float32 592B 14.0 24.0 ... 1.988e+03 2.011e+03 ... ... PSAL_ERROR (N_POINTS) float32 592B nan nan nan nan ... nan nan nan nan PSAL_QC (N_POINTS) int64 1kB 1 1 1 1 1 1 1 1 1 ... 1 1 1 1 1 1 1 1 TEMP (N_POINTS) float32 592B 23.4 23.35 23.33 ... 4.559 4.474 TEMP_ERROR (N_POINTS) float32 592B nan nan nan nan ... nan nan nan nan TEMP_QC (N_POINTS) int64 1kB 1 1 1 1 1 1 1 1 1 ... 1 1 1 1 1 1 1 1 TIME_QC (N_POINTS) int64 1kB 1 1 1 1 1 1 1 1 1 ... 1 1 1 1 1 1 1 1 Attributes: (8)
or all the profiles of a given float
ArgoSet = DataFetcher().float([6901254])
apDS = ArgoSet.load().data
Argopy gets all the data, including the metadata in a xarray format: very handy
apDS
<xarray.Dataset> Dimensions: (N_POINTS: 15786) Coordinates: * N_POINTS (N_POINTS) int64 0 1 2 3 4 ... 15782 15783 15784 15785 LATITUDE (N_POINTS) float64 29.16 29.16 29.16 ... 21.51 21.51 21.51 LONGITUDE (N_POINTS) float64 -15.49 -15.49 -15.49 ... -20.96 -20.96 TIME (N_POINTS) datetime64[ns] 2018-10-23T20:54:00 ... 2023-0... Data variables: (12/15) CYCLE_NUMBER (N_POINTS) int64 1 1 1 1 1 1 1 ... 165 165 165 165 165 165 DATA_MODE (N_POINTS) <U1 'R' 'R' 'R' 'R' 'R' ... 'R' 'R' 'R' 'R' 'R' DIRECTION (N_POINTS) <U1 'D' 'D' 'D' 'D' 'D' ... 'A' 'A' 'A' 'A' 'A' PLATFORM_NUMBER (N_POINTS) int64 6901254 6901254 ... 6901254 6901254 POSITION_QC (N_POINTS) int64 1 1 1 1 1 1 1 1 1 1 ... 1 1 1 1 1 1 1 1 1 PRES (N_POINTS) float64 14.0 24.0 34.0 ... 2.013e+03 2.03e+03 ... ... PSAL_ERROR (N_POINTS) float64 nan nan nan nan nan ... nan nan nan nan PSAL_QC (N_POINTS) int64 1 1 1 1 1 1 1 1 1 1 ... 1 1 1 1 1 1 1 1 1 TEMP (N_POINTS) float64 23.4 23.35 23.33 ... 3.814 3.759 3.715 TEMP_ERROR (N_POINTS) float64 nan nan nan nan nan ... nan nan nan nan TEMP_QC (N_POINTS) int64 1 1 1 1 1 1 1 1 1 1 ... 1 1 1 1 1 1 1 1 1 TIME_QC (N_POINTS) int64 1 1 1 1 1 1 1 1 1 1 ... 1 1 1 1 1 1 1 1 1 Attributes: (8)
- N_POINTS: 15786
- N_POINTS(N_POINTS)int640 1 2 3 ... 15782 15783 15784 15785
array([ 0, 1, 2, ..., 15783, 15784, 15785])
- LATITUDE(N_POINTS)float6429.16 29.16 29.16 ... 21.51 21.51
- _CoordinateAxisType :
- Lat
- actual_range :
- [19.454 31.479]
- axis :
- Y
- colorBarMaximum :
- 90.0
- colorBarMinimum :
- -90.0
- ioos_category :
- Location
- long_name :
- Latitude of the station, best estimate
- standard_name :
- latitude
- units :
- degrees_north
- valid_max :
- 90.0
- valid_min :
- -90.0
- casted :
- 1
array([29.162, 29.162, 29.162, ..., 21.51 , 21.51 , 21.51 ])
- LONGITUDE(N_POINTS)float64-15.49 -15.49 ... -20.96 -20.96
- _CoordinateAxisType :
- Lon
- actual_range :
- [-24.536 -13.851]
- axis :
- X
- colorBarMaximum :
- 180.0
- colorBarMinimum :
- -180.0
- ioos_category :
- Location
- long_name :
- Longitude of the station, best estimate
- standard_name :
- longitude
- units :
- degrees_east
- valid_max :
- 180.0
- valid_min :
- -180.0
- casted :
- 1
array([-15.488, -15.488, -15.488, ..., -20.956, -20.956, -20.956])
- TIME(N_POINTS)datetime64[ns]2018-10-23T20:54:00 ... 2023-03-...
- _CoordinateAxisType :
- Time
- actual_range :
- [1.54032804e+09 1.67937690e+09]
- axis :
- T
- ioos_category :
- Time
- long_name :
- Julian day (UTC) of the station relative to REFERENCE_DATE_TIME
- standard_name :
- time
- time_origin :
- 01-JAN-1970 00:00:00
- casted :
- 1
array(['2018-10-23T20:54:00.000000000', '2018-10-23T20:54:00.000000000', '2018-10-23T20:54:00.000000000', ..., '2023-03-21T05:35:00.000000000', '2023-03-21T05:35:00.000000000', '2023-03-21T05:35:00.000000000'], dtype='datetime64[ns]')
- CYCLE_NUMBER(N_POINTS)int641 1 1 1 1 1 ... 165 165 165 165 165
- long_name :
- Float cycle number
- conventions :
- 0..N, 0 : launch cycle (if exists), 1 : first complete cycle
- casted :
- 1
array([ 1, 1, 1, ..., 165, 165, 165])
- DATA_MODE(N_POINTS)<U1'R' 'R' 'R' 'R' ... 'R' 'R' 'R' 'R'
- long_name :
- Delayed mode or real time data
- conventions :
- R : real time; D : delayed mode; A : real time with adjustment
- casted :
- 1
array(['R', 'R', 'R', ..., 'R', 'R', 'R'], dtype='<U1')
- DIRECTION(N_POINTS)<U1'D' 'D' 'D' 'D' ... 'A' 'A' 'A' 'A'
- long_name :
- Direction of the station profiles
- conventions :
- A: ascending profiles, D: descending profiles
- casted :
- 1
array(['D', 'D', 'D', ..., 'A', 'A', 'A'], dtype='<U1')
- PLATFORM_NUMBER(N_POINTS)int646901254 6901254 ... 6901254 6901254
- long_name :
- Float unique identifier
- conventions :
- WMO float identifier : A9IIIII
- casted :
- 1
array([6901254, 6901254, 6901254, ..., 6901254, 6901254, 6901254])
- POSITION_QC(N_POINTS)int641 1 1 1 1 1 1 1 ... 1 1 1 1 1 1 1 1
- long_name :
- Global quality flag of POSITION_QC profile
- conventions :
- Argo reference table 2a
- casted :
- 1
array([1, 1, 1, ..., 1, 1, 1])
- PRES(N_POINTS)float6414.0 24.0 ... 2.013e+03 2.03e+03
- long_name :
- Sea Pressure
- standard_name :
- sea_water_pressure
- units :
- decibar
- valid_min :
- 0.0
- valid_max :
- 12000.0
- resolution :
- 0.1
- axis :
- Z
- casted :
- 1
array([ 14., 24., 34., ..., 1987., 2013., 2030.])
- PRES_ERROR(N_POINTS)float64nan nan nan nan ... nan nan nan nan
- long_name :
- ERROR IN Sea Pressure
- standard_name :
- sea_water_pressure
- units :
- decibar
- valid_min :
- 0.0
- valid_max :
- 12000.0
- resolution :
- 0.1
- axis :
- Z
- casted :
- 1
array([nan, nan, nan, ..., nan, nan, nan])
- PRES_QC(N_POINTS)int641 1 1 1 1 1 1 1 ... 1 1 1 1 1 1 1 1
- long_name :
- Global quality flag of PRES_QC profile
- conventions :
- Argo reference table 2a
- casted :
- 1
array([1, 1, 1, ..., 1, 1, 1])
- PSAL(N_POINTS)float6436.69 36.71 36.71 ... 35.01 35.01
- long_name :
- PRACTICAL SALINITY
- standard_name :
- sea_water_salinity
- units :
- psu
- valid_min :
- 0.0
- valid_max :
- 43.0
- resolution :
- 0.001
- casted :
- 1
array([36.69400024, 36.70500183, 36.70899963, ..., 35.01800156, 35.01300049, 35.00899887])
- PSAL_ERROR(N_POINTS)float64nan nan nan nan ... nan nan nan nan
- long_name :
- ERROR IN PRACTICAL SALINITY
- standard_name :
- sea_water_salinity
- units :
- psu
- valid_min :
- 0.0
- valid_max :
- 43.0
- resolution :
- 0.001
- casted :
- 1
array([nan, nan, nan, ..., nan, nan, nan])
- PSAL_QC(N_POINTS)int641 1 1 1 1 1 1 1 ... 1 1 1 1 1 1 1 1
- long_name :
- Global quality flag of PSAL_QC profile
- conventions :
- Argo reference table 2a
- casted :
- 1
array([1, 1, 1, ..., 1, 1, 1])
- TEMP(N_POINTS)float6423.4 23.35 23.33 ... 3.759 3.715
- long_name :
- SEA TEMPERATURE IN SITU ITS-90 SCALE
- standard_name :
- sea_water_temperature
- units :
- degree_Celsius
- valid_min :
- -2.0
- valid_max :
- 40.0
- resolution :
- 0.001
- casted :
- 1
array([23.40299988, 23.34799957, 23.33499908, ..., 3.81399989, 3.75900006, 3.71499991])
- TEMP_ERROR(N_POINTS)float64nan nan nan nan ... nan nan nan nan
- long_name :
- ERROR IN SEA TEMPERATURE IN SITU ITS-90 SCALE
- standard_name :
- sea_water_temperature
- units :
- degree_Celsius
- valid_min :
- -2.0
- valid_max :
- 40.0
- resolution :
- 0.001
- casted :
- 1
array([nan, nan, nan, ..., nan, nan, nan])
- TEMP_QC(N_POINTS)int641 1 1 1 1 1 1 1 ... 1 1 1 1 1 1 1 1
- long_name :
- Global quality flag of TEMP_QC profile
- conventions :
- Argo reference table 2a
- casted :
- 1
array([1, 1, 1, ..., 1, 1, 1])
- TIME_QC(N_POINTS)int641 1 1 1 1 1 1 1 ... 1 1 1 1 1 1 1 1
- long_name :
- Global quality flag of TIME_QC profile
- conventions :
- Argo reference table 2a
- casted :
- 1
array([1, 1, 1, ..., 1, 1, 1])
- DATA_ID :
- ARGO
- DOI :
- http://doi.org/10.17882/42182
- Fetched_from :
- https://erddap.ifremer.fr/erddap
- Fetched_by :
- pvb
- Fetched_date :
- 2024/08/21
- Fetched_constraints :
- WMO6901254
- Fetched_uri :
- ['https://erddap.ifremer.fr/erddap/tabledap/ArgoFloats.nc?data_mode,latitude,longitude,position_qc,time,time_qc,direction,platform_number,cycle_number,config_mission_number,vertical_sampling_scheme,pres,temp,psal,pres_qc,temp_qc,psal_qc,pres_adjusted,temp_adjusted,psal_adjusted,pres_adjusted_qc,temp_adjusted_qc,psal_adjusted_qc,pres_adjusted_error,temp_adjusted_error,psal_adjusted_error&platform_number=~"6901254"&distinct()&orderBy("time,pres")']
- history :
- Variables filtered according to DATA_MODE; Variables selected according to QC
note that the data is organized in ‘points’, a 1D array collection of measurements:
apDS.TEMP
<xarray.DataArray 'TEMP' (N_POINTS: 15786)> array([23.40299988, 23.34799957, 23.33499908, ..., 3.81399989, 3.75900006, 3.71499991]) Coordinates: * N_POINTS (N_POINTS) int64 0 1 2 3 4 5 ... 15781 15782 15783 15784 15785 LATITUDE (N_POINTS) float64 29.16 29.16 29.16 29.16 ... 21.51 21.51 21.51 LONGITUDE (N_POINTS) float64 -15.49 -15.49 -15.49 ... -20.96 -20.96 -20.96 TIME (N_POINTS) datetime64[ns] 2018-10-23T20:54:00 ... 2023-03-21T0... Attributes: (7)
- N_POINTS: 15786
- 23.4 23.35 23.33 23.0 20.89 19.76 ... 3.915 3.871 3.814 3.759 3.715
array([23.40299988, 23.34799957, 23.33499908, ..., 3.81399989, 3.75900006, 3.71499991])
- N_POINTS(N_POINTS)int640 1 2 3 ... 15782 15783 15784 15785
array([ 0, 1, 2, ..., 15783, 15784, 15785])
- LATITUDE(N_POINTS)float6429.16 29.16 29.16 ... 21.51 21.51
- _CoordinateAxisType :
- Lat
- actual_range :
- [19.454 31.479]
- axis :
- Y
- colorBarMaximum :
- 90.0
- colorBarMinimum :
- -90.0
- ioos_category :
- Location
- long_name :
- Latitude of the station, best estimate
- standard_name :
- latitude
- units :
- degrees_north
- valid_max :
- 90.0
- valid_min :
- -90.0
- casted :
- 1
array([29.162, 29.162, 29.162, ..., 21.51 , 21.51 , 21.51 ])
- LONGITUDE(N_POINTS)float64-15.49 -15.49 ... -20.96 -20.96
- _CoordinateAxisType :
- Lon
- actual_range :
- [-24.536 -13.851]
- axis :
- X
- colorBarMaximum :
- 180.0
- colorBarMinimum :
- -180.0
- ioos_category :
- Location
- long_name :
- Longitude of the station, best estimate
- standard_name :
- longitude
- units :
- degrees_east
- valid_max :
- 180.0
- valid_min :
- -180.0
- casted :
- 1
array([-15.488, -15.488, -15.488, ..., -20.956, -20.956, -20.956])
- TIME(N_POINTS)datetime64[ns]2018-10-23T20:54:00 ... 2023-03-...
- _CoordinateAxisType :
- Time
- actual_range :
- [1.54032804e+09 1.67937690e+09]
- axis :
- T
- ioos_category :
- Time
- long_name :
- Julian day (UTC) of the station relative to REFERENCE_DATE_TIME
- standard_name :
- time
- time_origin :
- 01-JAN-1970 00:00:00
- casted :
- 1
array(['2018-10-23T20:54:00.000000000', '2018-10-23T20:54:00.000000000', '2018-10-23T20:54:00.000000000', ..., '2023-03-21T05:35:00.000000000', '2023-03-21T05:35:00.000000000', '2023-03-21T05:35:00.000000000'], dtype='datetime64[ns]')
- long_name :
- SEA TEMPERATURE IN SITU ITS-90 SCALE
- standard_name :
- sea_water_temperature
- units :
- degree_Celsius
- valid_min :
- -2.0
- valid_max :
- 40.0
- resolution :
- 0.001
- casted :
- 1
However, and for the purpose of the Argo online school is easier to work with the data in profiles; argopy allows the transformation:
data=apDS.argo.point2profile()
data
<xarray.Dataset> Dimensions: (N_PROF: 165, N_LEVELS: 98) Coordinates: * N_PROF (N_PROF) int64 0 1 2 3 4 5 6 ... 159 160 161 162 163 164 * N_LEVELS (N_LEVELS) int64 0 1 2 3 4 5 6 7 ... 91 92 93 94 95 96 97 LATITUDE (N_PROF) float64 29.16 29.18 29.17 ... 20.89 21.23 21.51 LONGITUDE (N_PROF) float64 -15.49 -15.43 -15.38 ... -20.47 -20.96 TIME (N_PROF) datetime64[ns] 2018-10-23T20:54:00 ... 2023-03-... Data variables: (12/15) CYCLE_NUMBER (N_PROF) int64 1 1 2 3 4 5 6 ... 160 161 162 163 164 165 DATA_MODE (N_PROF) <U1 'R' 'R' 'R' 'R' 'R' ... 'R' 'R' 'R' 'R' 'R' DIRECTION (N_PROF) <U1 'D' 'A' 'A' 'A' 'A' ... 'A' 'A' 'A' 'A' 'A' PLATFORM_NUMBER (N_PROF) int64 6901254 6901254 6901254 ... 6901254 6901254 POSITION_QC (N_PROF) int64 1 1 1 1 1 1 1 1 1 1 ... 1 1 1 1 1 1 1 1 1 1 PRES (N_PROF, N_LEVELS) float64 14.0 24.0 ... 2.013e+03 2.03e+03 ... ... PSAL_ERROR (N_PROF) float64 nan nan nan nan nan ... nan nan nan nan PSAL_QC (N_PROF) int64 1 1 1 1 1 1 1 1 1 1 ... 1 1 1 1 1 1 1 1 1 1 TEMP (N_PROF, N_LEVELS) float64 23.4 23.35 23.33 ... 3.759 3.715 TEMP_ERROR (N_PROF) float64 nan nan nan nan nan ... nan nan nan nan TEMP_QC (N_PROF) int64 1 1 1 1 1 1 1 1 1 1 ... 1 1 1 1 1 1 1 1 1 1 TIME_QC (N_PROF) int64 1 1 1 1 1 1 1 1 1 1 ... 1 1 1 1 1 1 1 1 1 1 Attributes: (8)
- N_PROF: 165
- N_LEVELS: 98
- N_PROF(N_PROF)int640 1 2 3 4 5 ... 160 161 162 163 164
- casted :
- 1
array([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39, 40, 41, 42, 43, 44, 45, 46, 47, 48, 49, 50, 51, 52, 53, 54, 55, 56, 57, 58, 59, 60, 61, 62, 63, 64, 65, 66, 67, 68, 69, 70, 71, 72, 73, 74, 75, 76, 77, 78, 79, 80, 81, 82, 83, 84, 85, 86, 87, 88, 89, 90, 91, 92, 93, 94, 95, 96, 97, 98, 99, 100, 101, 102, 103, 104, 105, 106, 107, 108, 109, 110, 111, 112, 113, 114, 115, 116, 117, 118, 119, 120, 121, 122, 123, 124, 125, 126, 127, 128, 129, 130, 131, 132, 133, 134, 135, 136, 137, 138, 139, 140, 141, 142, 143, 144, 145, 146, 147, 148, 149, 150, 151, 152, 153, 154, 155, 156, 157, 158, 159, 160, 161, 162, 163, 164])
- N_LEVELS(N_LEVELS)int640 1 2 3 4 5 6 ... 92 93 94 95 96 97
- casted :
- 1
array([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39, 40, 41, 42, 43, 44, 45, 46, 47, 48, 49, 50, 51, 52, 53, 54, 55, 56, 57, 58, 59, 60, 61, 62, 63, 64, 65, 66, 67, 68, 69, 70, 71, 72, 73, 74, 75, 76, 77, 78, 79, 80, 81, 82, 83, 84, 85, 86, 87, 88, 89, 90, 91, 92, 93, 94, 95, 96, 97])
- LATITUDE(N_PROF)float6429.16 29.18 29.17 ... 21.23 21.51
- _CoordinateAxisType :
- Lat
- actual_range :
- [19.454 31.479]
- axis :
- Y
- colorBarMaximum :
- 90.0
- colorBarMinimum :
- -90.0
- ioos_category :
- Location
- long_name :
- Latitude of the station, best estimate
- standard_name :
- latitude
- units :
- degrees_north
- valid_max :
- 90.0
- valid_min :
- -90.0
- casted :
- 1
array([29.162, 29.182, 29.173, 29.146, 29.107, 29.076, 28.795, 28.503, 28.17 , 28.495, 28.73 , 28.813, 29.055, 29.31 , 28.756, 28.644, 29.159, 29.306, 28.919, 28.362, 28.223, 28.082, 27.974, 27.783, 27.773, 27.733, 27.635, 27.639, 27.856, 28.253, 28.087, 28.275, 28.662, 28.911, 29.116, 29.135, 29.231, 29.458, 29.697, 29.993, 30.171, 30.154, 30.097, 30.081, 30.21 , 30.307, 30.468, 30.587, 31.06 , 31.479, 31.097, 30.973, 30.708, 30.673, 30.654, 30.663, 30.161, 29.439, 29.401, 29.711, 30.359, 30.317, 29.441, 29.899, 29.412, 29.417, 28.903, 28.754, 28.318, 27.862, 27.541, 27.218, 27.034, 26.627, 26.517, 26.371, 26.115, 25.681, 25.245, 25.024, 25.098, 24.98 , 24.676, 24.571, 24.653, 24.779, 24.751, 24.804, 24.75 , 24.569, 24.299, 23.97 , 23.803, 23.935, 24.109, 24.507, 24.93 , 25.223, 24.976, 24.448, 24.375, 24.56 , 24.602, 24.314, 23.92 , 23.301, 23.158, 23.482, 23.758, 23.865, 24.199, 24.331, 24.416, 24.252, 24.096, 23.892, 23.657, 23.301, 22.93 , 22.568, 22.253, 22.077, 22.048, 22.201, 22.479, 23.032, 23.649, 23.988, 23.475, 22.84 , 22.552, 22.403, 22.528, 22.681, 22.883, 22.964, 22.899, 22.563, 22.149, 22.03 , 22.281, 22.729, 23.006, 23.046, 23.04 , 23.224, 23.691, 23.524, 23.137, 22.832, 22.507, 22.198, 21.893, 22.071, 22.136, 21.973, 21.527, 21.09 , 20.389, 19.605, 19.454, 20.016, 20.895, 21.228, 21.51 ])
- LONGITUDE(N_PROF)float64-15.49 -15.43 ... -20.47 -20.96
- _CoordinateAxisType :
- Lon
- actual_range :
- [-24.536 -13.851]
- axis :
- X
- colorBarMaximum :
- 180.0
- colorBarMinimum :
- -180.0
- ioos_category :
- Location
- long_name :
- Longitude of the station, best estimate
- standard_name :
- longitude
- units :
- degrees_east
- valid_max :
- 180.0
- valid_min :
- -180.0
- casted :
- 1
array([-15.488, -15.429, -15.381, -15.344, -15.31 , -15.271, -14.948, -14.94 , -15.203, -15.447, -15.661, -15.784, -15.803, -15.342, -15.165, -15.75 , -15.959, -15.562, -15.35 , -15.317, -15.329, -15.144, -15.007, -14.79 , -14.56 , -14.559, -14.844, -15.04 , -15.12 , -15.039, -15.122, -14.955, -14.846, -14.642, -14.475, -14.303, -14.091, -13.939, -13.851, -13.979, -14.123, -14.314, -14.462, -14.553, -14.708, -14.692, -14.835, -15.256, -15.729, -15.493, -15.741, -16.103, -16.638, -17.116, -17.51 , -17.845, -17.917, -18.501, -18.917, -19.45 , -19.585, -19.153, -19.436, -20.251, -19.673, -20.336, -20.014, -19.997, -20.16 , -20.636, -21.114, -21.552, -21.482, -21.736, -21.839, -21.676, -21.436, -21.212, -21.063, -20.968, -21.057, -21.459, -21.708, -21.759, -21.759, -21.886, -22.002, -22.133, -22.286, -22.283, -22.246, -22.047, -21.917, -22.038, -22.285, -22.686, -22.891, -22.745, -22.328, -22.125, -21.93 , -21.642, -21.323, -21.296, -21.217, -20.856, -20.336, -20.281, -20.473, -20.693, -21.089, -21.424, -21.671, -21.906, -22.136, -22.268, -22.409, -22.506, -22.503, -22.481, -22.431, -22.538, -22.733, -23.048, -23.508, -24.121, -24.536, -24.312, -23.725, -23.703, -23.723, -23.801, -23.773, -23.803, -23.877, -23.898, -23.681, -23.308, -22.901, -22.586, -22.436, -22.19 , -21.962, -21.849, -21.81 , -21.819, -21.383, -20.857, -20.766, -20.822, -20.791, -20.548, -20.134, -19.965, -19.597, -19.207, -19.071, -19.171, -19.738, -19.571, -19.192, -19.162, -19.809, -20.466, -20.956])
- TIME(N_PROF)datetime64[ns]2018-10-23T20:54:00 ... 2023-03-...
- _CoordinateAxisType :
- Time
- actual_range :
- [1.54032804e+09 1.67937690e+09]
- axis :
- T
- ioos_category :
- Time
- long_name :
- Julian day (UTC) of the station relative to REFERENCE_DATE_TIME
- standard_name :
- time
- time_origin :
- 01-JAN-1970 00:00:00
- casted :
- 1
array(['2018-10-23T20:54:00.000000000', '2018-10-25T05:30:00.000000000', '2018-10-27T05:30:00.000000000', '2018-10-29T05:24:00.000000000', '2018-10-31T05:28:00.000000000', '2018-11-02T05:32:00.000000000', '2018-11-12T05:34:00.000000000', '2018-11-22T05:26:00.000000000', '2018-12-02T05:37:00.000000000', '2018-12-12T05:35:00.000000000', '2018-12-22T05:33:00.000000000', '2019-01-01T05:26:00.000000000', '2019-01-11T05:29:00.000000000', '2019-01-21T05:29:00.000000000', '2019-01-31T05:35:00.000000000', '2019-02-10T05:29:00.000000000', '2019-02-20T05:43:00.000000000', '2019-03-02T05:36:00.000000000', '2019-03-12T05:37:00.000000000', '2019-03-22T05:33:00.000000000', '2019-04-01T05:26:00.000000000', '2019-04-11T05:33:00.000000000', '2019-04-21T05:42:00.000000000', '2019-05-01T05:36:00.000000000', '2019-05-11T05:32:00.000000000', '2019-05-21T05:34:00.000000000', '2019-05-31T05:41:00.000000000', '2019-06-10T05:27:00.000000000', '2019-06-20T05:39:00.000000000', '2019-06-30T05:42:00.000000000', '2019-07-10T05:40:00.000000000', '2019-07-20T05:35:00.000000000', '2019-07-30T05:35:00.000000000', '2019-08-09T05:37:00.000000000', '2019-08-19T05:47:00.000000000', '2019-08-29T05:25:00.000000000', '2019-09-08T05:24:00.000000000', '2019-09-18T05:29:00.000000000', '2019-09-28T05:26:00.000000000', '2019-10-08T05:25:00.000000000', ... '2022-02-24T05:24:00.000000000', '2022-03-06T05:22:00.000000000', '2022-03-16T05:12:00.000000000', '2022-03-26T05:18:00.000000000', '2022-04-05T04:59:00.000000000', '2022-04-15T05:16:00.000000000', '2022-04-25T05:23:00.000000000', '2022-05-05T05:25:00.000000000', '2022-05-15T05:24:00.000000000', '2022-05-25T05:09:00.000000000', '2022-06-04T05:31:00.000000000', '2022-06-14T05:20:00.000000000', '2022-06-24T05:11:00.000000000', '2022-07-04T05:07:00.000000000', '2022-07-14T05:26:00.000000000', '2022-07-24T05:23:00.000000000', '2022-08-03T05:20:00.000000000', '2022-08-13T04:54:00.000000000', '2022-08-23T05:26:00.000000000', '2022-09-02T05:29:00.000000000', '2022-09-12T05:24:00.000000000', '2022-09-22T05:25:00.000000000', '2022-10-02T05:23:00.000000000', '2022-10-12T05:17:00.000000000', '2022-10-22T05:13:00.000000000', '2022-11-01T05:15:00.000000000', '2022-11-21T05:11:00.000000000', '2022-12-01T05:11:00.000000000', '2022-12-11T05:13:00.000000000', '2022-12-21T05:15:00.000000000', '2022-12-31T05:22:00.000000000', '2023-01-10T05:23:00.000000000', '2023-01-20T05:14:00.000000000', '2023-01-30T05:15:00.000000000', '2023-02-09T05:12:00.000000000', '2023-02-19T05:15:00.000000000', '2023-03-01T05:06:00.000000000', '2023-03-11T05:26:00.000000000', '2023-03-21T05:35:00.000000000'], dtype='datetime64[ns]')
- CYCLE_NUMBER(N_PROF)int641 1 2 3 4 5 ... 161 162 163 164 165
- long_name :
- Float cycle number
- conventions :
- 0..N, 0 : launch cycle (if exists), 1 : first complete cycle
- casted :
- 1
array([ 1, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39, 40, 41, 42, 43, 44, 45, 46, 47, 48, 49, 50, 51, 52, 53, 54, 55, 56, 57, 58, 59, 60, 61, 62, 63, 64, 65, 66, 67, 68, 69, 70, 71, 72, 73, 74, 75, 76, 77, 78, 79, 80, 81, 82, 83, 84, 85, 86, 87, 88, 89, 90, 91, 92, 93, 94, 95, 96, 97, 98, 99, 100, 101, 102, 103, 104, 105, 106, 107, 108, 109, 110, 111, 112, 113, 114, 115, 116, 117, 118, 119, 120, 121, 122, 123, 124, 125, 126, 127, 128, 129, 130, 131, 132, 133, 134, 135, 136, 137, 138, 139, 140, 141, 142, 143, 144, 145, 146, 147, 148, 149, 150, 151, 153, 154, 155, 156, 157, 158, 159, 160, 161, 162, 163, 164, 165])
- DATA_MODE(N_PROF)<U1'R' 'R' 'R' 'R' ... 'R' 'R' 'R' 'R'
- long_name :
- Delayed mode or real time data
- conventions :
- R : real time; D : delayed mode; A : real time with adjustment
- casted :
- 1
array(['R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R', 'R'], dtype='<U1')
- DIRECTION(N_PROF)<U1'D' 'A' 'A' 'A' ... 'A' 'A' 'A' 'A'
- long_name :
- Direction of the station profiles
- conventions :
- A: ascending profiles, D: descending profiles
- casted :
- 1
array(['D', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A', 'A'], dtype='<U1')
- PLATFORM_NUMBER(N_PROF)int646901254 6901254 ... 6901254 6901254
- long_name :
- Float unique identifier
- conventions :
- WMO float identifier : A9IIIII
- casted :
- 1
array([6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254, 6901254])
- POSITION_QC(N_PROF)int641 1 1 1 1 1 1 1 ... 1 1 1 1 1 1 1 1
- long_name :
- Global quality flag of POSITION_QC profile
- conventions :
- Argo reference table 2a
- casted :
- 1
array([1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1])
- PRES(N_PROF, N_LEVELS)float6414.0 24.0 ... 2.013e+03 2.03e+03
- long_name :
- Sea Pressure
- standard_name :
- sea_water_pressure
- units :
- decibar
- valid_min :
- 0.0
- valid_max :
- 12000.0
- resolution :
- 0.1
- axis :
- Z
- casted :
- 1
array([[ 14., 24., 34., ..., nan, nan, nan], [ 6., 7., 8., ..., 1988., 2011., nan], [ 6., 7., 8., ..., 1989., nan, nan], ..., [ 6., 7., 8., ..., 1982., nan, nan], [ 6., 7., 8., ..., 1988., 2007., nan], [ 6., 7., 8., ..., 1987., 2013., 2030.]])
- PRES_ERROR(N_PROF)float64nan nan nan nan ... nan nan nan nan
- long_name :
- ERROR IN Sea Pressure
- standard_name :
- sea_water_pressure
- units :
- decibar
- valid_min :
- 0.0
- valid_max :
- 12000.0
- resolution :
- 0.1
- axis :
- Z
- casted :
- 1
array([nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan])
- PRES_QC(N_PROF)int641 1 1 1 1 1 1 1 ... 1 1 1 1 1 1 1 1
- long_name :
- Global quality flag of PRES_QC profile
- conventions :
- Argo reference table 2a
- casted :
- 1
array([1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1])
- PSAL(N_PROF, N_LEVELS)float6436.69 36.71 36.71 ... 35.01 35.01
- long_name :
- PRACTICAL SALINITY
- standard_name :
- sea_water_salinity
- units :
- psu
- valid_min :
- 0.0
- valid_max :
- 43.0
- resolution :
- 0.001
- casted :
- 1
array([[36.69400024, 36.70500183, 36.70899963, ..., nan, nan, nan], [36.6969986 , 36.6969986 , 36.6969986 , ..., 35.15299988, 35.14199829, nan], [36.68999863, 36.68999863, 36.68999863, ..., 35.1570015 , nan, nan], ..., [36.01200104, 36.01100159, 36.01100159, ..., 35.00899887, nan, nan], [36.27999878, 36.27899933, 36.27799988, ..., 35.01200104, 35.01200104, nan], [36.15000153, 36.15000153, 36.15100098, ..., 35.01800156, 35.01300049, 35.00899887]])
- PSAL_ERROR(N_PROF)float64nan nan nan nan ... nan nan nan nan
- long_name :
- ERROR IN PRACTICAL SALINITY
- standard_name :
- sea_water_salinity
- units :
- psu
- valid_min :
- 0.0
- valid_max :
- 43.0
- resolution :
- 0.001
- casted :
- 1
array([nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan])
- PSAL_QC(N_PROF)int641 1 1 1 1 1 1 1 ... 1 1 1 1 1 1 1 1
- long_name :
- Global quality flag of PSAL_QC profile
- conventions :
- Argo reference table 2a
- casted :
- 1
array([1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1])
- TEMP(N_PROF, N_LEVELS)float6423.4 23.35 23.33 ... 3.759 3.715
- long_name :
- SEA TEMPERATURE IN SITU ITS-90 SCALE
- standard_name :
- sea_water_temperature
- units :
- degree_Celsius
- valid_min :
- -2.0
- valid_max :
- 40.0
- resolution :
- 0.001
- casted :
- 1
array([[23.40299988, 23.34799957, 23.33499908, ..., nan, nan, nan], [23.41200066, 23.41200066, 23.41399956, ..., 4.55900002, 4.47399998, nan], [23.4090004 , 23.40600014, 23.40800095, ..., 4.579 , nan, nan], ..., [19. , 19.00099945, 19.00200081, ..., 3.82399988, nan, nan], [19.74099922, 19.73900032, 19.72400093, ..., 3.83999991, 3.8210001 , nan], [20.20999908, 20.21100044, 20.20599937, ..., 3.81399989, 3.75900006, 3.71499991]])
- TEMP_ERROR(N_PROF)float64nan nan nan nan ... nan nan nan nan
- long_name :
- ERROR IN SEA TEMPERATURE IN SITU ITS-90 SCALE
- standard_name :
- sea_water_temperature
- units :
- degree_Celsius
- valid_min :
- -2.0
- valid_max :
- 40.0
- resolution :
- 0.001
- casted :
- 1
array([nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan, nan])
- TEMP_QC(N_PROF)int641 1 1 1 1 1 1 1 ... 1 1 1 1 1 1 1 1
- long_name :
- Global quality flag of TEMP_QC profile
- conventions :
- Argo reference table 2a
- casted :
- 1
array([1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1])
- TIME_QC(N_PROF)int641 1 1 1 1 1 1 1 ... 1 1 1 1 1 1 1 1
- long_name :
- Global quality flag of TIME_QC profile
- conventions :
- Argo reference table 2a
- casted :
- 1
array([1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1])
- DATA_ID :
- ARGO
- DOI :
- http://doi.org/10.17882/42182
- Fetched_from :
- https://erddap.ifremer.fr/erddap
- Fetched_by :
- pvb
- Fetched_date :
- 2024/08/21
- Fetched_constraints :
- WMO6901254
- Fetched_uri :
- ['https://erddap.ifremer.fr/erddap/tabledap/ArgoFloats.nc?data_mode,latitude,longitude,position_qc,time,time_qc,direction,platform_number,cycle_number,config_mission_number,vertical_sampling_scheme,pres,temp,psal,pres_qc,temp_qc,psal_qc,pres_adjusted,temp_adjusted,psal_adjusted,pres_adjusted_qc,temp_adjusted_qc,psal_adjusted_qc,pres_adjusted_error,temp_adjusted_error,psal_adjusted_error&platform_number=~"6901254"&distinct()&orderBy("time,pres")']
- history :
- Variables filtered according to DATA_MODE; Variables selected according to QC; Transformed with point2profile
The core-Argo profile files contain the core parameters provided by a float: pressure, temperature, salinity, conductivity (PRES, TEMP, PSAL, CNDC).
Now, let’s see how this argopy data set works as a xarray, and it easy to plot any data. Let’s begin ploting the first two profiles:
fig, ax = plt.subplots(1, 2, figsize=(8,10), sharey=True)
#Temperature
ax[0].plot(data.TEMP[0],-data.PRES[0],'ro',label='N_PROF=0 D Fetcher')
ax[0].plot(data.TEMP[1],-data.PRES[1],'bo',label='N_PROF=1 A Fetcher')
ax[0].set_title(data['TEMP'].attrs['long_name'])
ax[0].set_ylabel(data['PRES'].attrs['long_name'])
ax[0].grid()
ax[0].legend();
ax[1].plot(data.PSAL[0],-data.PRES[0],'ro',label='N_PROF=0 D Fetcher')
ax[1].plot(data.PSAL[1],-data.PRES[1],'bo',label='N_PROF=1 A Fetcher')
ax[1].set_title(data['TEMP'].attrs['long_name'])
ax[1].grid()
ax[1].legend();
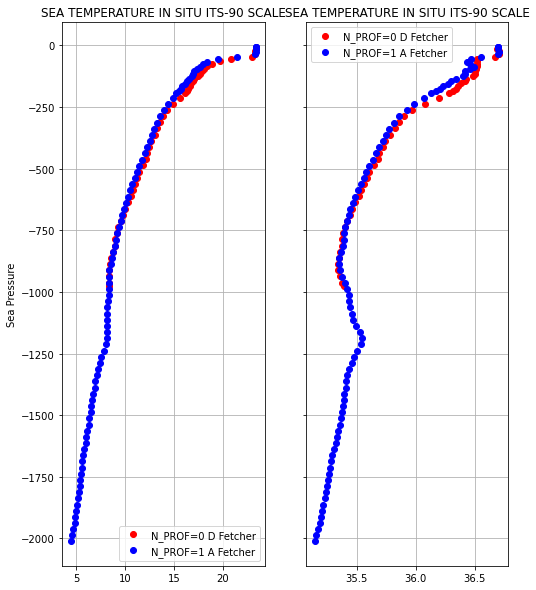
and of course the locations of these two profiles and the rest of them:
fig, ax = plt.subplots(figsize=(8,8))
ax.plot(data.LONGITUDE[0],data.LATITUDE[0],'ks',markersize=10,label='001D N_PROF=0 Descending')
ax.plot(data.LONGITUDE[1],data.LATITUDE[1],'bs',markersize=10,label='001 N_PROF=0 Ascending')
ax.plot(data.LONGITUDE[2:],data.LATITUDE[2:],'bo',label='rest of the proiles')
ax.grid()
ax.legend();
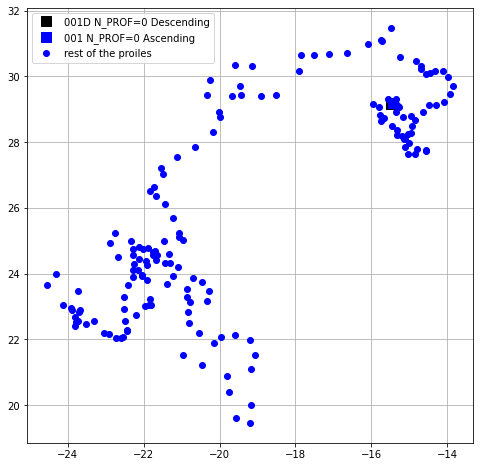
or using the plotting routines of argopy, easyly we can plot the full trayectory
scatter_map(data, set_global=False);
/Users/pvb/miniconda3/envs/AOS/lib/python3.9/site-packages/argopy/plot/plot.py:409: UserWarning: More than one N_LEVELS found in this dataset, scatter_map will use the first level only
warnings.warn("More than one N_LEVELS found in this dataset, scatter_map will use the first level only")
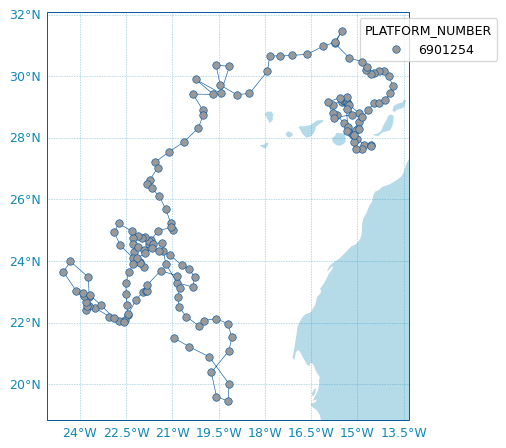
argopy is powerfull with continous updates, an de refer to the argopy Documentation for a more detailled explanation of its capabilities.